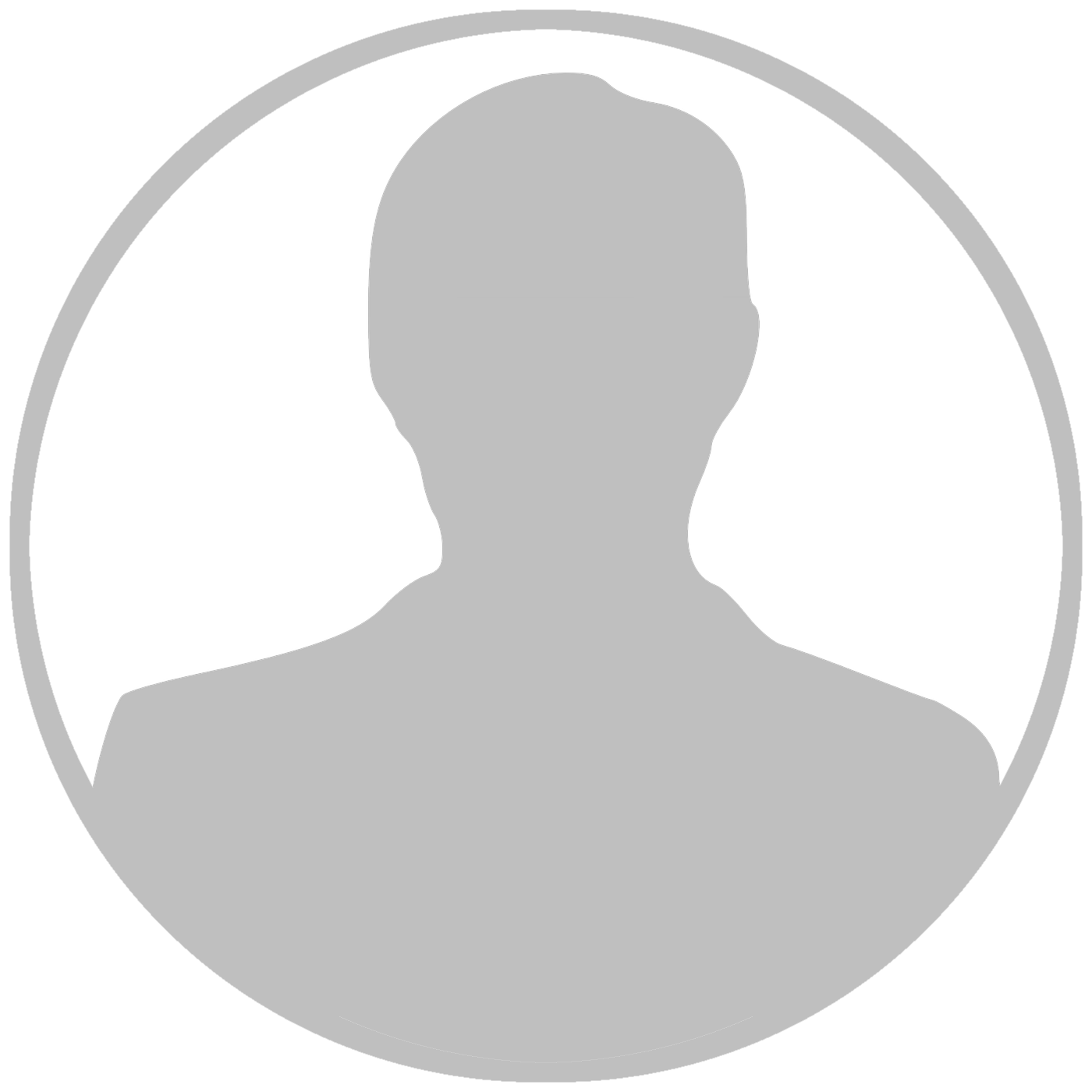
Ever since Android 9+ switched to Conscrypt we can no longer efficiently encrypt (and decrypt) large files with AES-GCM. We did’t notice this before because when using 16 byte IVs even modern Androids will fall back to bouncy castle. However the 'bug'/'feature' in Conscrypt surfaced when we switched over to 12 byte IVs (which uses Conscrypt on Android 9+) Switching back entirely to 16 byte IVs is undesirable as this would break compatibility with Monal. So we end up with a weird compromise where we use 12 byte for normale plain text OMEMO messages and 'small' files where the inefficiencies aren’t a problem. The result of this commit is that Monal won’t be able to receive our files larger than 768KiB. However the alternative is that Conversations would always OOM when attempting to send larger files (where large depends on the available RAM.) fixes #3653
256 lines
8.1 KiB
Groovy
256 lines
8.1 KiB
Groovy
// Top-level build file where you can add configuration options common to all
|
|
// sub-projects/modules.
|
|
buildscript {
|
|
repositories {
|
|
google()
|
|
jcenter()
|
|
}
|
|
dependencies {
|
|
classpath 'com.android.tools.build:gradle:3.5.3'
|
|
}
|
|
}
|
|
|
|
apply plugin: 'com.android.application'
|
|
|
|
repositories {
|
|
google()
|
|
jcenter()
|
|
mavenCentral()
|
|
}
|
|
|
|
configurations {
|
|
playstoreImplementation
|
|
compatImplementation
|
|
conversationsFreeCompatImplementation
|
|
conversationsPlaystoreCompatImplementation
|
|
conversationsPlaystoreSystemImplementation
|
|
quicksyFreeCompatImplementation
|
|
quicksyImplementation
|
|
}
|
|
|
|
ext {
|
|
supportLibVersion = '28.0.0'
|
|
}
|
|
|
|
dependencies {
|
|
playstoreImplementation('com.google.firebase:firebase-messaging:17.3.4') {
|
|
exclude group: 'com.google.firebase', module: 'firebase-core'
|
|
exclude group: 'com.google.firebase', module: 'firebase-analytics'
|
|
exclude group: 'com.google.firebase', module: 'firebase-measurement-connector'
|
|
}
|
|
conversationsPlaystoreCompatImplementation("com.android.installreferrer:installreferrer:1.1")
|
|
conversationsPlaystoreSystemImplementation("com.android.installreferrer:installreferrer:1.1")
|
|
implementation 'org.sufficientlysecure:openpgp-api:10.0'
|
|
implementation('com.theartofdev.edmodo:android-image-cropper:2.7.+') {
|
|
exclude group: 'com.android.support', module: 'appcompat-v7'
|
|
exclude group: 'com.android.support', module: 'exifinterface'
|
|
}
|
|
implementation "com.android.support:support-v13:$supportLibVersion"
|
|
implementation "com.android.support:appcompat-v7:$supportLibVersion"
|
|
implementation "com.android.support:exifinterface:$supportLibVersion"
|
|
implementation "com.android.support:cardview-v7:$supportLibVersion"
|
|
implementation "com.android.support:support-emoji:$supportLibVersion"
|
|
implementation "com.android.support:design:$supportLibVersion"
|
|
compatImplementation "com.android.support:support-emoji-appcompat:$supportLibVersion"
|
|
conversationsFreeCompatImplementation "com.android.support:support-emoji-bundled:$supportLibVersion"
|
|
quicksyFreeCompatImplementation "com.android.support:support-emoji-bundled:$supportLibVersion"
|
|
implementation 'org.bouncycastle:bcmail-jdk15on:1.58'
|
|
//zxing stopped supporting Java 7 so we have to stick with 3.3.3
|
|
//https://github.com/zxing/zxing/issues/1170
|
|
implementation 'com.google.zxing:core:3.3.3'
|
|
implementation 'de.measite.minidns:minidns-hla:0.2.4'
|
|
implementation 'me.leolin:ShortcutBadger:1.1.22@aar'
|
|
implementation 'org.whispersystems:signal-protocol-java:2.6.2'
|
|
implementation 'com.makeramen:roundedimageview:2.3.0'
|
|
implementation "com.wefika:flowlayout:0.4.1"
|
|
implementation 'net.ypresto.androidtranscoder:android-transcoder:0.3.0'
|
|
implementation project(':libs:xmpp-addr')
|
|
implementation 'org.osmdroid:osmdroid-android:6.1.5'
|
|
implementation 'org.hsluv:hsluv:0.2'
|
|
implementation 'org.conscrypt:conscrypt-android:2.2.1'
|
|
implementation 'me.drakeet.support:toastcompat:1.1.0'
|
|
implementation "com.leinardi.android:speed-dial:2.0.1"
|
|
//retrofit needs to stick with 2.6.x (https://github.com/square/retrofit/blob/master/CHANGELOG.md)
|
|
implementation "com.squareup.retrofit2:retrofit:2.6.4"
|
|
implementation "com.squareup.retrofit2:converter-gson:2.6.4"
|
|
//okhttp needs to stick with 3.12.x
|
|
implementation 'com.squareup.okhttp3:okhttp:3.12.7'
|
|
implementation 'com.google.guava:guava:27.1-android'
|
|
quicksyImplementation 'io.michaelrocks:libphonenumber-android:8.11.1'
|
|
}
|
|
|
|
ext {
|
|
travisBuild = System.getenv("TRAVIS") == "true"
|
|
preDexEnabled = System.getProperty("pre-dex", "true")
|
|
}
|
|
|
|
android {
|
|
compileSdkVersion 29
|
|
|
|
defaultConfig {
|
|
minSdkVersion 16
|
|
targetSdkVersion 28
|
|
versionCode 365
|
|
versionName "2.7.1"
|
|
archivesBaseName += "-$versionName"
|
|
applicationId "eu.siacs.conversations"
|
|
resValue "string", "applicationId", applicationId
|
|
resValue "string", "app_name", "Conversations"
|
|
buildConfigField "String", "LOGTAG", "\"conversations\""
|
|
}
|
|
|
|
dataBinding {
|
|
enabled true
|
|
}
|
|
|
|
dexOptions {
|
|
// Skip pre-dexing when running on Travis CI or when disabled via -Dpre-dex=false.
|
|
preDexLibraries = preDexEnabled && !travisBuild
|
|
jumboMode true
|
|
}
|
|
|
|
compileOptions {
|
|
sourceCompatibility JavaVersion.VERSION_1_8
|
|
targetCompatibility JavaVersion.VERSION_1_8
|
|
}
|
|
|
|
flavorDimensions("mode", "distribution", "emoji")
|
|
|
|
productFlavors {
|
|
|
|
quicksy {
|
|
dimension "mode"
|
|
applicationId = "im.quicksy.client"
|
|
resValue "string", "app_name", "Quicksy"
|
|
resValue "string", "applicationId", applicationId
|
|
buildConfigField "String", "LOGTAG", "\"quicksy\""
|
|
}
|
|
|
|
conversations {
|
|
dimension "mode"
|
|
}
|
|
|
|
playstore {
|
|
dimension "distribution"
|
|
versionNameSuffix "+p"
|
|
}
|
|
free {
|
|
dimension "distribution"
|
|
versionNameSuffix "+f"
|
|
}
|
|
system {
|
|
dimension "emoji"
|
|
versionNameSuffix "s"
|
|
}
|
|
compat {
|
|
dimension "emoji"
|
|
versionNameSuffix "c"
|
|
}
|
|
}
|
|
|
|
sourceSets {
|
|
quicksyFreeCompat {
|
|
java {
|
|
srcDir 'src/freeCompat/java'
|
|
}
|
|
}
|
|
quicksyPlaystoreCompat {
|
|
java {
|
|
srcDir 'src/playstoreCompat/java'
|
|
}
|
|
res {
|
|
srcDir 'src/playstoreCompat/res'
|
|
srcDir 'src/quicksyPlaystore/res'
|
|
}
|
|
}
|
|
quicksyPlaystoreSystem {
|
|
res {
|
|
srcDir 'src/quicksyPlaystore/res'
|
|
}
|
|
}
|
|
conversationsFreeCompat {
|
|
java {
|
|
srcDir 'src/freeCompat/java'
|
|
srcDir 'src/conversationsFree/java'
|
|
}
|
|
}
|
|
conversationsFreeSystem {
|
|
java {
|
|
srcDir 'src/conversationsFree/java'
|
|
}
|
|
}
|
|
conversationsPlaystoreCompat {
|
|
java {
|
|
srcDir 'src/playstoreCompat/java'
|
|
srcDir 'src/conversationsPlaystore/java'
|
|
}
|
|
res {
|
|
srcDir 'src/playstoreCompat/res'
|
|
srcDir 'src/conversationsPlaystore/res'
|
|
}
|
|
}
|
|
conversationsPlaystoreSystem {
|
|
java {
|
|
srcDir 'src/conversationsPlaystore/java'
|
|
}
|
|
res {
|
|
srcDir 'src/conversationsPlaystore/res'
|
|
}
|
|
}
|
|
}
|
|
|
|
buildTypes {
|
|
release {
|
|
shrinkResources true
|
|
minifyEnabled true
|
|
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
|
|
versionNameSuffix "r"
|
|
}
|
|
debug {
|
|
shrinkResources true
|
|
minifyEnabled true
|
|
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
|
|
versionNameSuffix "d"
|
|
}
|
|
}
|
|
|
|
|
|
if (new File("signing.properties").exists()) {
|
|
Properties props = new Properties()
|
|
props.load(new FileInputStream(file("signing.properties")))
|
|
|
|
signingConfigs {
|
|
release {
|
|
storeFile file(props['keystore'])
|
|
storePassword props['keystore.password']
|
|
keyAlias props['keystore.alias']
|
|
keyPassword props['keystore.password']
|
|
}
|
|
}
|
|
buildTypes.release.signingConfig = signingConfigs.release
|
|
}
|
|
|
|
lintOptions {
|
|
disable 'ExtraTranslation', 'MissingTranslation', 'InvalidPackage', 'MissingQuantity', 'AppCompatResource'
|
|
}
|
|
|
|
subprojects {
|
|
|
|
afterEvaluate {
|
|
if (getPlugins().hasPlugin('android') ||
|
|
getPlugins().hasPlugin('android-library')) {
|
|
|
|
configure(android.lintOptions) {
|
|
disable 'AndroidGradlePluginVersion', 'MissingTranslation'
|
|
}
|
|
}
|
|
|
|
}
|
|
}
|
|
|
|
packagingOptions {
|
|
exclude 'META-INF/BCKEY.DSA'
|
|
exclude 'META-INF/BCKEY.SF'
|
|
}
|
|
}
|